Here is an example of how to build the bottom navigation bar.
NavigationBar is included in flutter/material.dart
package.
This widget allows toggle between your screens.
Structure is:
Scaffold( bottomNavigationBar: NavigationBar( onDestinationSelected: ..., selectedIndex: _currentPageIndex, destinations: ..., ), body: ... );
How it looks:
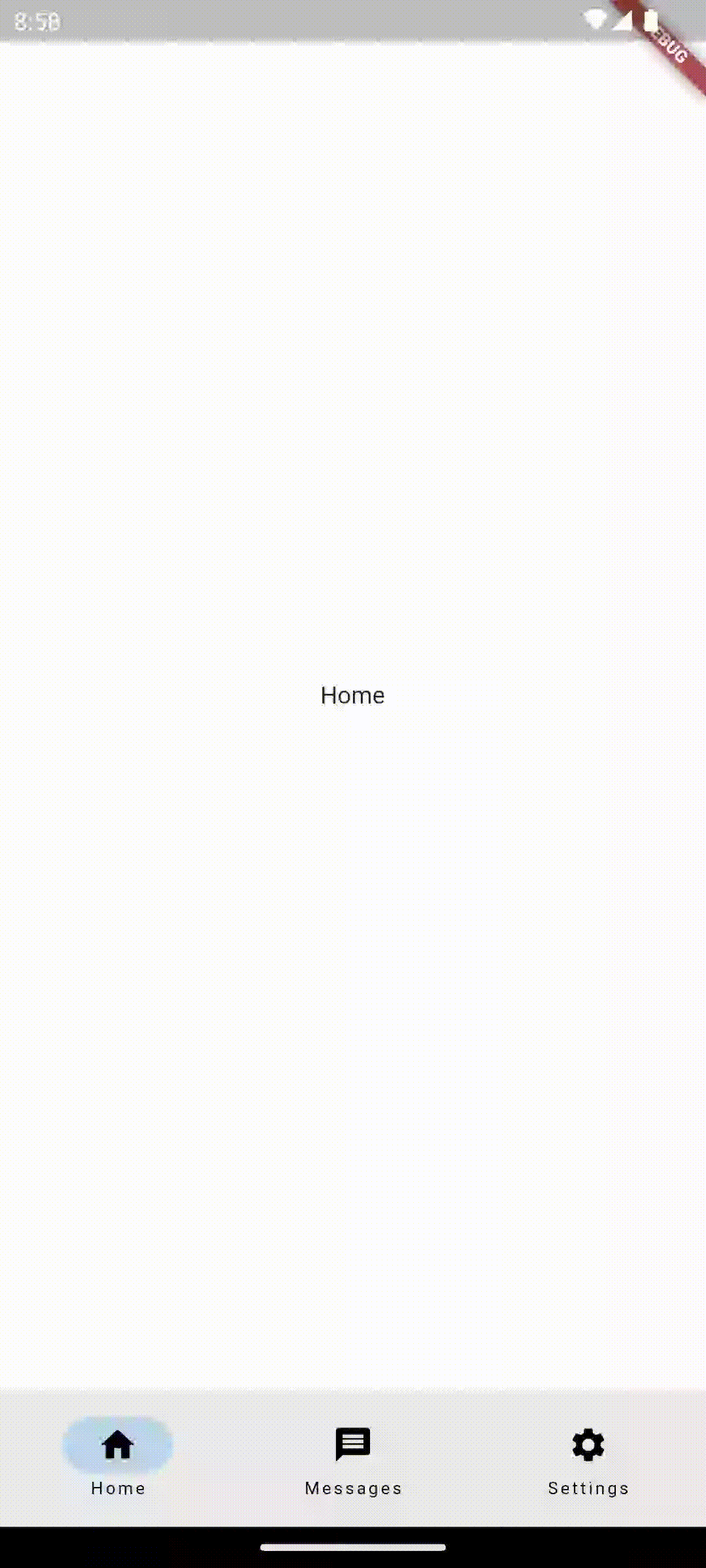
Full code example:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { WidgetsFlutterBinding.ensureInitialized(); return const MaterialApp( home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key}); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int _currentPageIndex = 0; @override Widget build(BuildContext context) { return Scaffold( bottomNavigationBar: NavigationBar( onDestinationSelected: (int index) { setState(() { _currentPageIndex = index; }); }, selectedIndex: _currentPageIndex, destinations: const <Widget>[ NavigationDestination( icon: Icon(Icons.home), label: 'Home', ), NavigationDestination( icon: Icon(Icons.message), label: 'Messages', ), NavigationDestination( icon: Icon(Icons.settings), label: 'Settings', ), ], ), body: <Widget>[ Container( color: Colors.white, alignment: Alignment.center, child: const Text('Home'), ), Container( color: Colors.amberAccent, alignment: Alignment.center, child: const Text('Messages'), ), Container( color: Colors.cyanAccent, alignment: Alignment.center, child: const Text('Settings'), ), ][_currentPageIndex], ); } }